using EzySlice;
void FixedUpdate()
{
RaycastHit hit;
var position = StartSlicingPoint.position;
Vector3 dir = EndSlicingPoint.position - position;
bool hasHit = Physics.Raycast(position, dir, out hit, dir.magnitude, Sliciable);
if (hasHit && VelocityEstimator.GetVelocityEstimate().magnitude > NeedVelocityPower)
{
if (hit.transform.gameObject.layer == LayerMask.NameToLayer("Bomb"))
{
// Play game over
m_AudioSource.PlayOneShot(SlicingClip[1]);
hit.transform.gameObject.SetActive(false);
FruitGameManager.Instance.GameOver();
return;
}
Slice(hit.transform.gameObject, hit.point, VelocityEstimator.GetVelocityEstimate());
}
}
void Slice(GameObject target, Vector3 planePosition, Vector3 slicerVelocity)
{
var position = StartSlicingPoint.position;
Vector3 dir = EndSlicingPoint.position - position;
Vector3 planeNormal = Vector3.Cross(slicerVelocity, dir);
SlicedHull hull = target.Slice(planePosition, planeNormal, slicedMaterial);
if (hull != null)
{
GameObject upperHull = hull.CreateUpperHull(target, slicedMaterial);
GameObject lowerHull = hull.CreateLowerHull(target, slicedMaterial);
FruitGameManager.Instance.AddScore(1);
m_AudioSource.PlayOneShot(SlicingClip[0]);
CreatSlicedComponent(upperHull);
CreatSlicedComponent(lowerHull);
pool.ReturnObjectToPool(target);
}
}
void CreatSlicedComponent(GameObject slicedHull)
{
Rigidbody rb = slicedHull.AddComponent<Rigidbody>();
MeshCollider ms = slicedHull.AddComponent<MeshCollider>();
ms.convex = true;
rb.AddExplosionForce(slicedObjectInitVelocity *1.5f, slicedHull.transform.position, 1);
Destroy(slicedHull, 4f);
}
📋
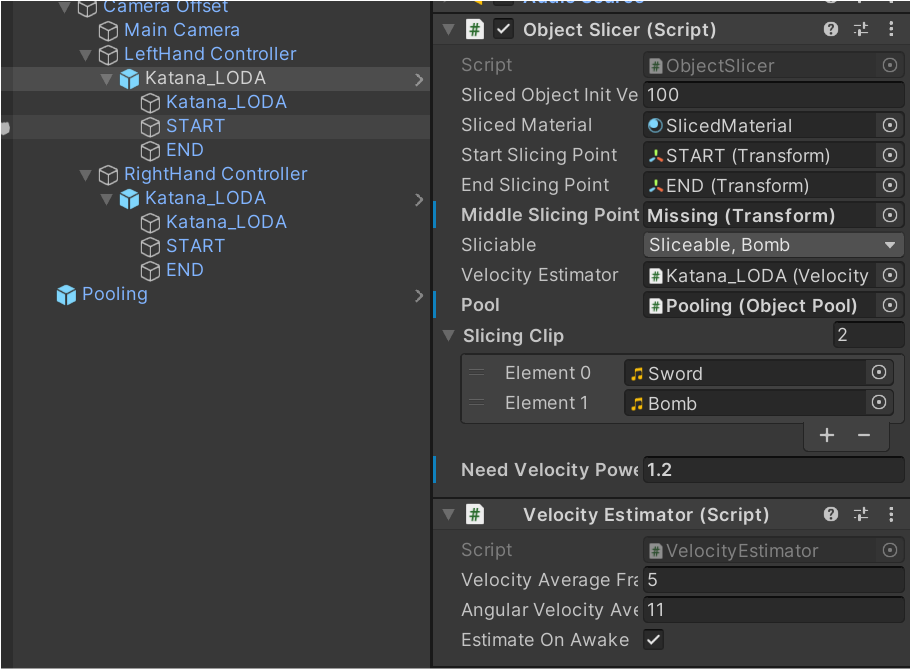